Mastering Complex App States in React: A Deep Dive into Redux and Context API
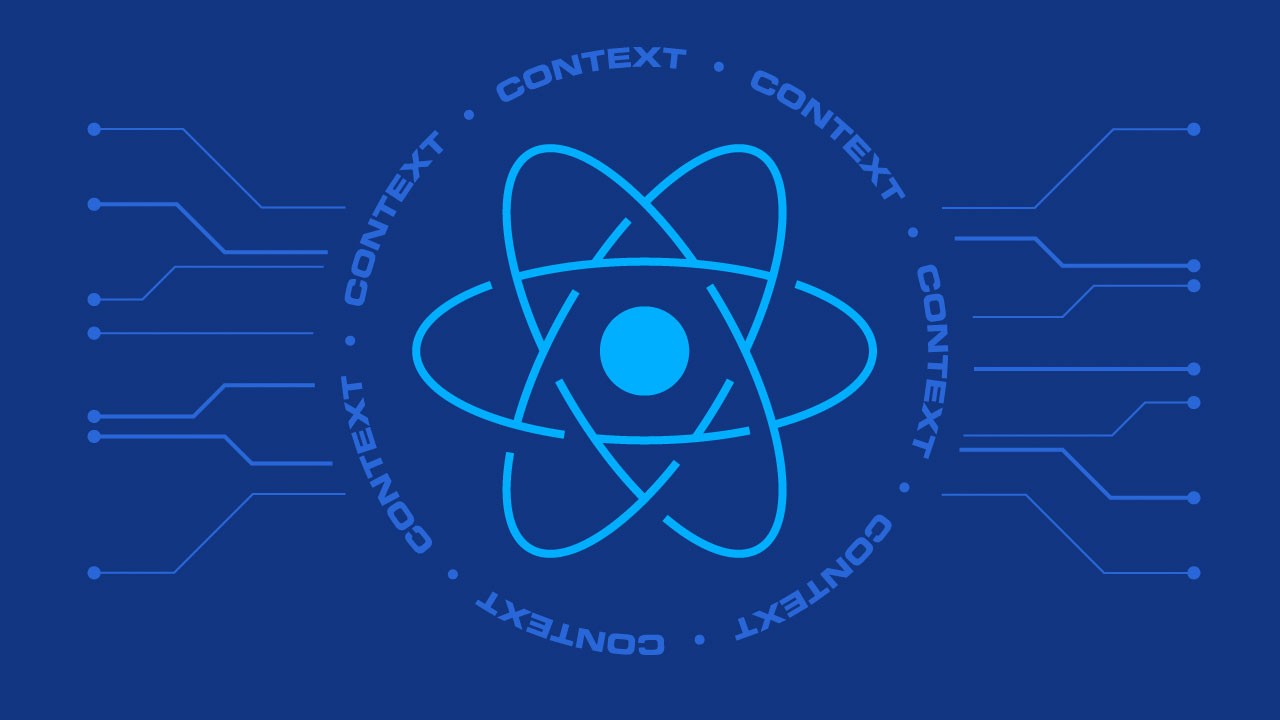
Effective state management in React is vital when you’re building applications. It shapes your app’s behavior and how users interact with it. Complex apps with lots of components interacting with the state can be hard to manage. This piece unpacks how to handle intricate app states in React using Redux and the Context API. We’ll also offer concrete examples to help illustrate each point.
Grasping React Application State Fundamentals
Known for crafting user interfaces, React is a leading JavaScript library. It’s particularly great for single-page apps where speed and user experiences are key. The ‘state’ is what fuels React’s ability to create dynamic, interactive user interfaces. Picture the state as a data pool, with a predetermined value when a component is ‘mounted’ or created. As users navigate your app, state values shift, enabling a dynamic experience. Mastering the management of state is vital for complicated apps with a diverse range of components that interact with the state. Appropriate state management in React can lead to easier-to-manage code and improved user experiences as components render and behave predictably. So, let’s go further and unravel the secrets of state manipulation in React.
Understanding Redux: An Overview and Its Value
From the thriving open-source community comes Redux, a clever JavaScript library designed for managing app state. It works well with React and isn’t limited to it, making it flexible for similar libraries too. Redux is defined by its unique structure of a centralized store, a secure storage that holds the entire app state. All components can access this unified store, allowing smooth communication and interaction. Redux gives you a predictable state container, ensuring clear, trackable, and manageable state changes. Why is Redux so crucial for managing complicated state in React? It’s all about simplicity and predictability. It simplifies state management by having a single truth source, doing away with the need for prop drilling, and making the state predictable with stringent rules about update methods. Its potent debugging tools and capacity to manage large app states establishes its role in state management. Digging deeper into Redux, we find effective ways to utilize this library to enhance state management in intricate React apps.
Using Redux: Real-World Application Examples
Picture an online shopping app where buyers pick products for their carts. Using Redux, we would create a central store as the state’s heart for the shopping cart. This store would hold an array, representing the items in the cart. Moreover, we would list actions that describe possible user activities – for example, adding or taking an item from the cart.
Imagine a user action prompted by a Redux dispatch. What follows is a process where a function called a ‘reducer’ computes the new state in a linear, methodical manner. The process flows logically: an action launches, the reducer mines the existing state and the launched action to formulate a new state, and finally, we have an update in the store with this new state.
For instance, a user wants to buy a pair of sneakers. The action ‘ADD_TO_CART’ launches, armed with the sneaker’s product details. Watching this action, the shopping cart reducer adds the sneakers to the current state’s item list, making a new state possessing the added sneakers. Consequently, the Redux store amends the shopping cart state, showcasing the added sneakers.
Therefore, Redux uses a systematic approach to manage complex state transitions in React. It offers a way to track state changes, making the application’s behavior more predictable and debugging simpler. Plus, it enables state management and manipulation from any part of your app, enhancing flexibility and user experience.
Getting Familiar with React’s Context API
React’s Context API is a stellar feature designed for efficient state management across convoluted component trees. At its core, the Context API allows parent components to share specific values with their descendants without manual prop passing. Picture how handy this could be if you had data – like a theme setting or user status – that needed to be accessible globally within your app.
The Context API was established in React 16.3. It has two main components: the Provider and the Consumer. The Provider wraps traditionally at the top, enclosing the parts needing the context values. The Consumer is used by parts wishing to use these shared values.
The Context API takes care of prop drilling. No need for other state management libraries for sharing basic state. But, there are limits to the Context API. It’s not for managing complex or frequent updates. Here though, Redux has its golden moment. It bridges the strengths of Redux with the simplicity of Context API. This fusion creates a powerful state management system in your app. Next, we will see some practical examples of how to use Redux and Context API together.
Unpacking the Context API with Practical Examples
Let’s use a real-world example to understand React’s Context API. Pretend you’re making a React app with a theme-switching feature. Here, the Context API saves the day. It offers a method to spread the current theme (light or dark) and a toggle for the theme across all parts in the app.
Start by enclosing your top component’s children in a Provider component. Pass both the current theme and the toggle function as values. Doing this forms a context, giving these values reachable to all child parts wrapped in this Provider.
So, you’ll use the Consumer component to obtain values in a child component. This tool snags the current theme and theme-altering function without the need to shuttle props through the component tree. Voila, any child component can flip easily between light and dark themes. This allows a tailored and changing experience for users.
Note, the Context API is good for sharing basic states, but it has some issues with many or updated states. Here, using Redux with the Context API really works well. More on that upcoming, promise! See how these mighty tools can boost your state management.
Merging Redux and Context API for Better State Management
Pairing Redux and the Context API can supercharge your app’s state management, making a solid, flexible system. Redux adds its strong foundation, awesome for dealing with detailed states and dealing with actions happening at different times. At the same time, the Context API makes sure data is easily accessed across all components. This means no need to pass props. The partnership between Redux and Context API makes managing complex state transitions easier and shared data quickly available. So, it enhances your experience as a developer and the app functionality. Using these libraries combined allows for effective handling of various state management situations. It maintains neat code and predictable app behavior. This combination not only improves state management but also boosts user experience by ensuring consistent and top-notch app performance. Time to dive in, explore, and see the bigger benefits of dealing with complex app states in React by linking Redux and the Context API.
Using Redux and Context API Together: Real Life Examples
Picture this. You’re building an online store that can speak many languages. Redux is great for tracking products and items in a shopping cart. The Context API, on the other hand, is effortless for remembering language choices and makes it easy for the whole app to use this information.
Suppose a shopper from France puts something in their cart. The system records ‘ADD_TO_CART’ and all the product info. Redux then makes sure everything is updated as it’s supposed to be. At the same time, the whole app knows to show everything in French thanks to the Context API. Redux and Context API work great together to make online shopping smooth for everyone, no matter what language they prefer.
So, with a little bit of code, we have a smooth-running system. This shows how well Redux and Context API work together. Redux takes care of complex changes while Context API makes shared information accessible to everyone. This helps us manage everything, keeps our code clean, and ensures the app behaves as expected.
This is how Redux and Context API join forces. They bring their strengths to the table and offer a flexible system for managing complex situations in React. Give them a try in your next React project. You won’t be disappointed!
Transform your ideas into reality with our React Js development services.
Wrap Up
The world of web development keeps changing, and handling an intricate React app’s status can be hard. However, powerful tools like Redux and the Context API are here to assist. Redux provides a stable framework ensuring predictability and consistency, especially for intricate applications. In contrast, the Context API promotes easy sharing of status across many components, helping to avoid props drilling and boosting accessibility of the global status.
When combined, these two prove powerful. Redux skillfully copes with complicated status transitions, while the Context API makes shared status data easily accessible where it’s needed. By combining Redux’s sturdiness with the Context API’s simplicity, you create an endurable, flexible system. This not only improves app performance, enhancing user experience, but also keeps coding clean and the app’s behaviour predictable, making the developer’s task easier.
But remember, getting the hang of these tools needs practice and time. So, get on it, start using Redux and the Context API in your next React project. Always explore and learn. This will soon have you ready to handle any complex status management problem with elegance and efficiency. Enjoy your coding!
Frequently Asked Questions
1. What does ‘state’ mean in React?
In React, ‘state’ is a built-in tool. It lets its components shape and control their own information. It retains data values across different function calls and re-renderings of components.
2. How is managing state vital in React?
State management plays a big role in React. It molds the performance of your application and the user’s interaction. It ensures components work as they should, leading to easy-to-handle code.
3. Explain Redux and its role in handling state in React?
Redux is a tool in JavaScript for managing application state. Its centralized store carries the whole state of an application. It provides uniformity in state alterations by following strict rules on change methods.
4. Can Redux function with libraries other than React?
Indeed, Redux cooperates well with React but isn’t limited to it. It can function with other similar tools too.
5. What refers to the Context API in React?
The Context API in React intends to distribute specific parent component values to its offsprings without manually passing props at each level.
6. When to pick Context API over Redux?
Redux shines in managing intricate state, whereas, Context API excels in sharing simple state. Things like user login status or a theme setting can be shared across components using Context API.
7. Redux and Context API – can they function together?
Indeed, merging Redux and the Context API can greatly help when dealing with complex app states. Redux tackles detailed states and asynchronous actions. On the other hand, the Context API aids in the smooth flow of data throughout all components.
8. What’s Redux’s strategy for managing state changes?
Redux follows a systematic, one-way data journey. There’s a dispatch of an action, then a reducer calculates the fresh state based on that action and the current state, and finally, the store gets a state update.
9. Has the Context API the capacity to deal with complex transitions similar to Redux?
The functionality of the Context API isn’t optimal for overseeing sophisticated or frequent state modifications. It’s more suited for spreading simple state across components.
10. Is it required to learn state management thoroughly for making apps with React?
Absolutely, gaining solid knowledge of state management in React is crucial when it comes to creating intricate apps. It enhances the ease of code maintenance and guarantees that components perform and display as anticipated.